Open roasting project (3) [DIY]
This article is currently being written. I’m including some reference photos and code snippets in case you want to get a head start.
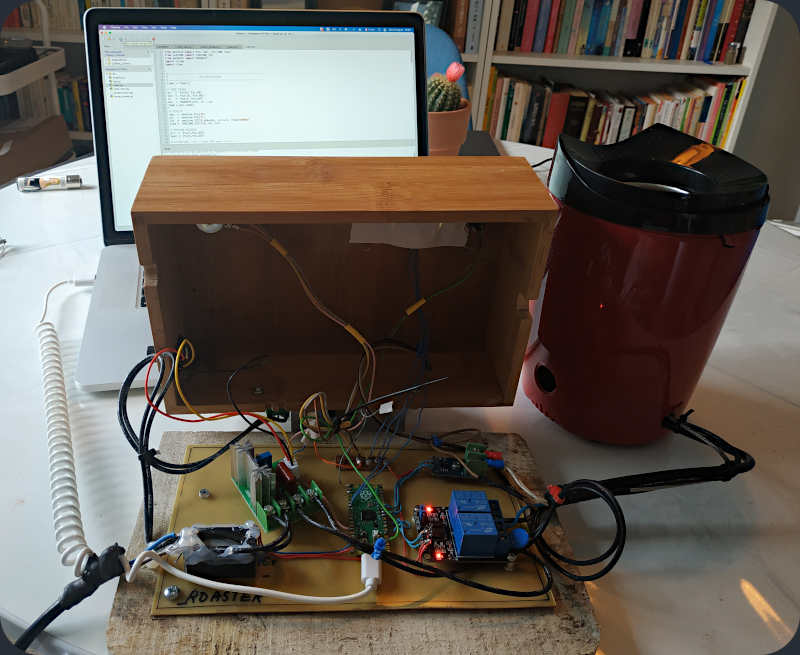
Introduction
This is the third part of the “Coffee Roaster” series. Here are the link to the first and second posts.
After this lengthy article, you will be able to move forward on your own and robotize everything you can think of. It’s all about getting data (from a sensor, a button, or whatever) and triggering things; while coordinating it all with a program.
The first step, for those of us who have no experience with PICO, is to follow a first tutorial to get started. I suggest this one: Starting with Raspberry PICO
Alright, now your PICO is micropythonized. We all are ready to go.
About the LED, the button and breadboard
Almost all the “2nd step kind of” tutorials will teach you how to turn on (or blink) an LED and use a strange four-legged button. ALL OF THEM! WHY??
Furthermore, the whole thing will be stuck in a breadboard that prevents you from visualise the reality of their electrical connections. So let’s get more creative and use the opportunity to learn about raspberry pico to roast coffee with proper soldering!
IMO, breadboards are useful for making quick connections and prototyping ideas. But for learning, it’s not ideal because it doesn’t look like the electronic schematic you may refer to (not to mention the fact that the connections are clumsy and come off in the slightest wind). In the electronics club of my youth, we used to attach a copper wire at the top (positive pole) and one at the bottom (for ground) to small wooden boards. Between these two wires, we could solder all of our elements the exact same way we would have them on a schematic.
The GPIOs
As you may have read somewhere, the Pico offers a number of pins. Some of them provide current (3.3V),others are ground pins (the square ones) and the rest are general purpose I/O (GPIO) that can be used to communicate to or from the Pico.
Here is the localisation of each component of device:
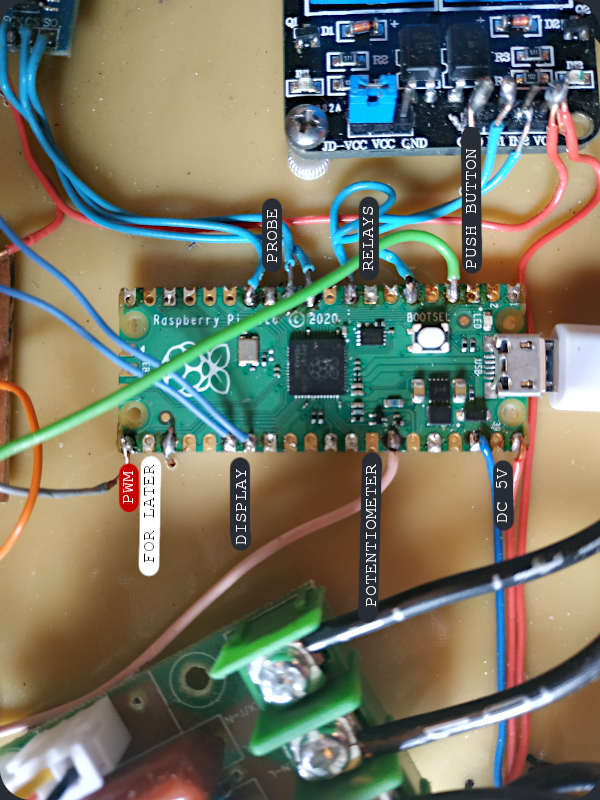
the start button
To start the roasting process, we need to push a nice, human sized, button. Any two pins button will do the job. It’s just a contact that is made which allows the current to flow. One leg will be connected to the current, while the second will be connected to one of the GPIOs (it will be defined as an input by our program). As it acts as a contact when pressed, the current will pass. By detecting it, we can trigger an action.
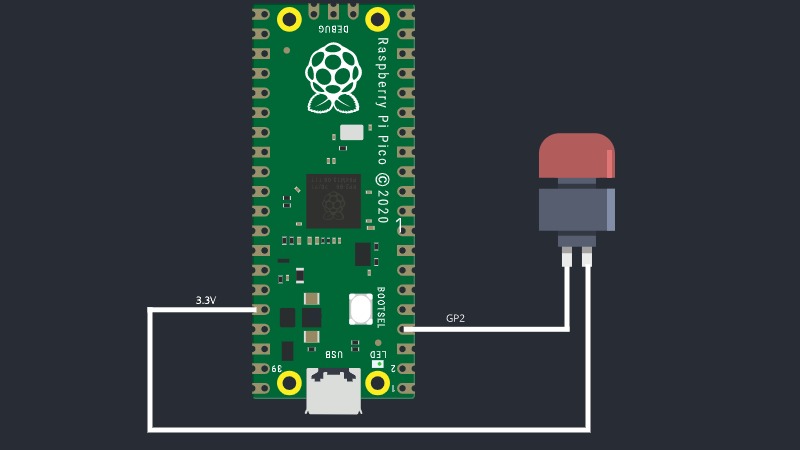
Here’s a short code to test your button
1 | from machine import Pin |
- First line: we import the general library that helps us deal with our PICO (here, to use the GPIOs)
- 2nd line: we create a variable that contains what the pico gets at the pin 2.
- Third: we create an infinite LOOP
- Fourth : (we are inside the loop) we detect if the button is pushed (the value of the variable “push” becomes 0)
- Fifth: (we are now within the condition that the button is pushed) we print something on the screen
It works but - as you can see - the code runs so fast that a simple push trigger many times our action. It doesn’t matter for now.
My explanations are very short. I apologise for that. For the purpose of this guide, I hope the provided information will be enough - for those with no coding background - to follow the theory and to experiment with the code.
the potentiometer
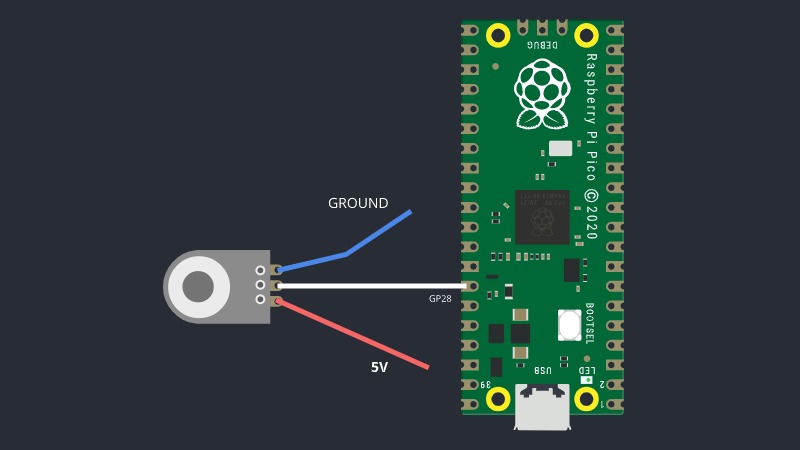
Here’s a simple code to test the potentiometer:
1 | pot = ADC(28) |
Here is a more substantial piece of code that takes over the preset selection of the roaster:
1 | pot = ADC(28) |
The temperature probe
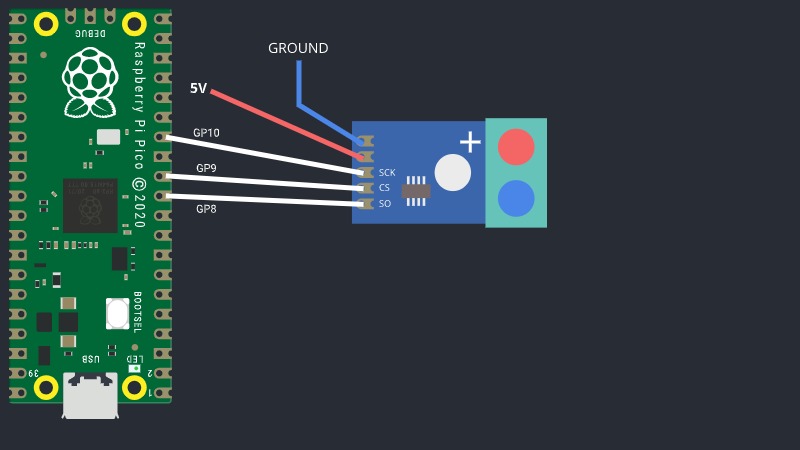
Library to install: max6675
Here’s a code to test the temperature probe:
1 | from max6675 import MAX6675 from machine import Pin |
The solid State Relays
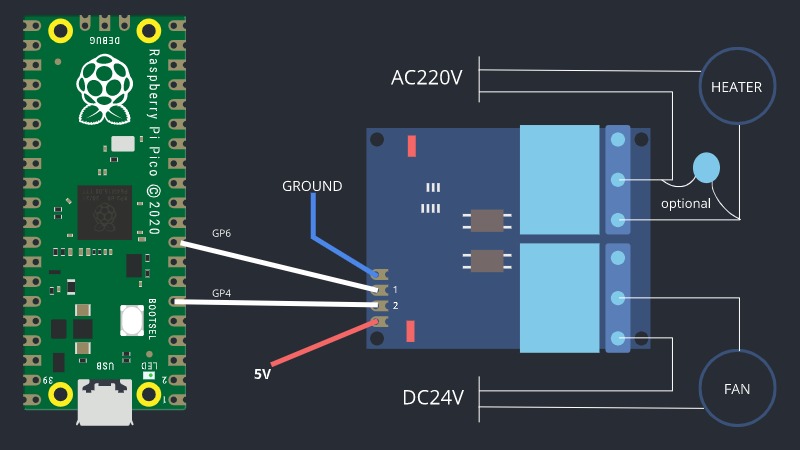
Simple Start / Stop functions that turn ON/OFF the heater and the fan’s relays. You can try it even without having connected anything to the relays. You will hear a click and the LEDs should light up.
1 | def start(): |
Note : As you can see, I added a component between the two poles of the heating resistor, at the output of the relay. It’s a varistor and its role is to limit the current under a certain voltage. Here, the value of the limit is not so important (mine is 270V). You can take something similar. Normally, the varistor is not stressed but when a voltage threshold is crossed (in my case 270V), it deflects the current to ground and thus protects the rest of the circuit (in this case, our heating resistor).Indeed, it can happen, when a relay is activated, that a very high voltage peak (of a few milliseconds only) is generated and potentially fries everything around.
You can find one here for example. Or anywhere really.
The oled screen
An oled screen is a lovely little display, very bright and very easy to set up. Mine is 128 by 64 pixels, which is enough for the roaster. You can find them everywhere. I got mine on Amazon for a few dollars.
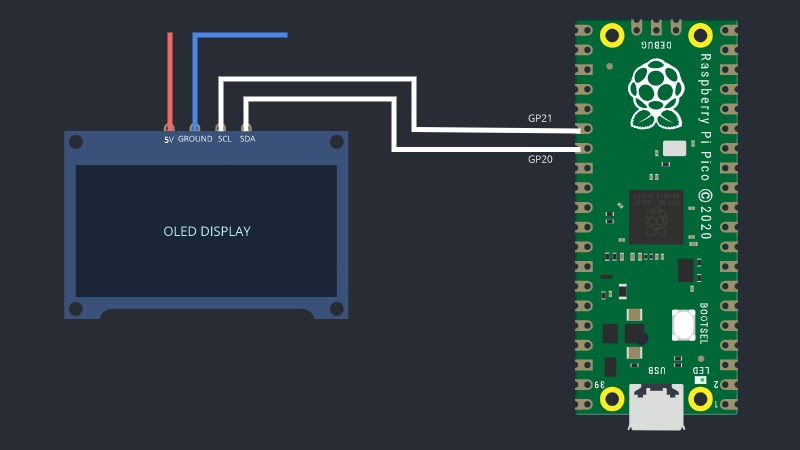
Library to install: ssd1306
Here’s a simple program to test the display:
1 | from machine import Pin, I2C |
Conclusion of the third part
Today, we have finally made good progress. The essentials are in place and we are now able to implement the start button, the display, the potentiometer, the relays and the temperature sensor.
In the next post, we’ll quickly see how to power the fan & heater and the PWM (to modulate the voltage that power the heater). Then our device will be finished. After that, all we need to do is code the program to run it all. That will be our fifth and last episode.
Take good care, good luck and see you presto!